Hosting and Implementing Widgets
This document provides detailed instructions on how to host and implement Moneyhub widgets, either on the Moneyhub platform or on your own site. Additionally, it covers the steps required to embed and configure widgets within your application.
Host
Hosted by Moneyhub
Widgets are automatically accessible on the Moneyhub platform. Once created, you don't need to take any additional steps to make them available.
Self-hosted
To host the widget on your own infrastructure, ensure your site is listed in the widget’s authorised domains. This is crucial for the widget to function correctly on your site and can be configured in the authorised domains section on the widget page. Configure this in the authorised domains section on the widget page.
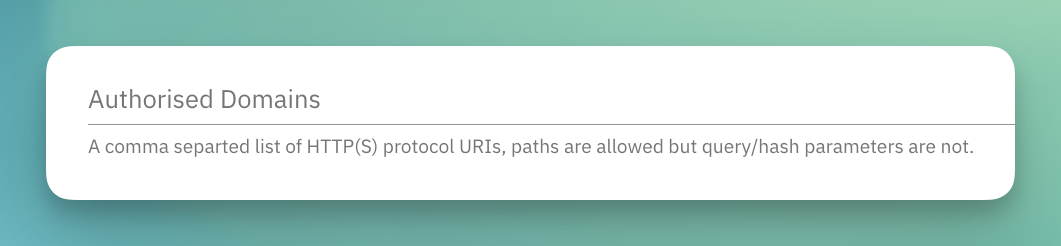
Implement
After creating a widget in the Admin Portal, you can view and utilise the code snippet to embed the widget into your site. Please follow these steps to ensure successful integration:
-
View Code Snippet:
Once the widget is created, an action to "View Code Snippet" will be available in the Admin Portal. Click this to access the snippet. -
Embed the Code Snippet:
Include the provided snippet in the<body>
of your HTML page. Here’s an example of how your code might look:<html> <head> <meta name="apple-mobile-web-app-capable" content="yes"> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <meta name="viewport" content="width=device-width, initial-scale=1, maximum-scale=1, user-scalable=no, minimal-ui"> </head> <body> <div id="root"></div> <script src="https://widgets.moneyhub.co.uk/widgets.bundle.js"></script> <script>window.moneyhub.init({ "elementId":"root", "type":"affordability", "widgetId":"defccf60-648e-41f2-9e2b-648010589956", "params":{ "externalUserId":"external-user-id", "email":"[email protected]", "name":"Custom name" } }) </script> </body> </html>
The example above illustrates a straightforward implementation where one of our widgets is initialised directly on page load. However, widgets can also be rendered using any JavaScript framework, such as React or Angular.
Event Listeners
Currently supported widgets: Affordability, Single Payment Widget
If you wish to log events that occur within the widget for your own diagnostic or auditing purposes, you can pass in a logger
object. This object should include functions named info
and/or error
, depending on the levels of events you want to capture.
When an event occurs, the widget will pass two arguments to these functions:
Argument | Description |
---|---|
Page Name | The name of the widget page where the event occurred. |
Data Object | A data object containing relevant information about the event. |
Example:
<html>
<head>
<meta name="apple-mobile-web-app-capable" content="yes">
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1, maximum-scale=1, user-scalable=no, minimal-ui">
</head>
<body>
<div id="root"></div>
<script src="https://widgets.moneyhub.co.uk/widgets.bundle.js"></script>
<script>window.moneyhub.init({
"elementId":"root",
"type":"affordability",
"widgetId":"defccf60-648e-41f2-9e2b-648010589956",
"params":{
"externalUserId":"external-user-id",
"email":"[email protected]",
"name":"Custom name"
},
"logger": {
// Replace with however you wish to handle these events
"info": (page, data) => console.info(page, data),
// Replace with however you wish to handle these events
"error": (page, data) => console.error(page, data),
}
})
</script>
</body>
</html>
By implementing this, you can capture and handle events within the widget efficiently, aiding in better logging and troubleshooting.
Updated 3 months ago